如何编写程序界面 通过最基本的方式去实现界面,编写XML代码,当掌握了使用XML来编写界面的方法之后,不管是进行高复杂度界面实现还是分析和修改当前现有的界面,都将手到擒来。
常见控件使用 新建一个UIWidgetTest项目,允许Android Studio自动创建活动,活动名和布局都使用默认值。
TextView TextView可以说是Android中最简单的空间了,它主要用于在界面上显示一段文本信息。 在res目录下创建一个layout目录,然后在其中创建一个activity_main.xml文件,往里面放置一个TextView。
1 2 3 4 5 6 7 8 9 10 11 12 <LinearLayout xmlns:android ="http://schemas.android.com/apk/res/android" android:orientation ="vertical" android:layout_width ="match_parent" android:layout_height ="match_parent" > <TextView android:id ="@+id/textView" android:layout_width ="match_parent" android:layout_height ="wrap_content" android:text ="TextView" /> </LinearLayout >
外层的LinearLayout(根元素)先忽略不看,在TextView中使用android:id给当前控件定义了一个唯一标识符。然后使用android:layout_width和android:layout_height指定控件的宽度和高度。Android中的所有控件的都有这两个属性,可选值有三种:match_parent、fill_parent和wrap_content,其中match_parent和fill_parent的功能相同。fill_parent是Android早期版本使用的属性,现在官方推荐使用match_parent,它的作用是让当前控件的大小和父布局的大小一样,也就是由父布局来决定当前控件的大小。wrap_content表示让当前控件的大小能够刚好包含里面的内容,也就是由控件内容决定当前控件的大小。 指定文字内容可以正常显示,但是TextView的宽度和屏幕是一样宽的。这是由于TextView中的文字默认是居左上角对其的,TextView的宽度充满了整个屏幕,由于文字内容不够长,所以效果不明显。我们可以修改文字对齐方式:
1 2 3 4 5 6 7 8 9 10 11 12 <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" android:orientation="vertical" android:layout_width="match_parent" android:layout_height="match_parent"> <TextView android:id="@+id/textView" android:layout_width="match_parent" android:layout_height="wrap_content" android:gravity="center" android:text="TextView" /> </LinearLayout>
我们还可以对TextVIew中文字的大小和颜色进行修改:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" android:orientation="vertical" android:layout_width="match_parent" android:layout_height="match_parent"> <TextView android:id="@+id/textView" android:layout_width="match_parent" android:layout_height="wrap_content" android:gravity="center" android:textSize="24sp" //指定大小 android:textColor="#00ff00" //指定颜色 android:text="TextView" /> </LinearLayout>
Button是程序用于和用户进行交互的一个重要控件,它的可配置的属性和TextView差不多,在activity_main.xml中加入Button:
1 2 3 4 5 6 7 8 9 10 11 <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" android:orientation="vertical" android:layout_width="match_parent" android:layout_height="match_parent"> ... <Button android:id="@+id/button" android:layout_width="match_parent" android:layout_height="wrap_content" android:text="Button" /> </LinearLayout>
而这里,布局文件里的文字是“Button”,但是最终的显示结果确实“BUTTON”。这是由于系统对Button中的所有英文字母自动进行了大写转换,我们可以禁用这一默认特性:
1 2 3 4 5 6 <Button android:id ="@+id/button" android:layout_width ="match_parent" android:layout_height ="wrap_content" android:text ="Button" android:textAllCaps ="false" />
然后在MainActivity中为Button的点击事件注册一个监听器:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 public class MainActivity extends AppCompatActivity { @Override protected void onCreate (@Nullable Bundle savedInstanceState) { super .onCreate(savedInstanceState); setContentView(R.layout.activity_main); Button button1 = (Button) findViewById(R.id.button); button1.setOnClickListener(new View .OnClickListener() { @Override public void onClick (View v) { } }); } }
当每次点击按钮时,就会执行监听器中的onClick()方法,我们只需要在方法中加入要处理的逻辑即可。如果不喜欢使用匿名类的方式来注册监听器,也可以使用实现接口的方式来注册:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 public class MainActivity extends AppCompatActivity implements View .OnClickListener{ @Override protected void onCreate (@Nullable Bundle savedInstanceState) { super .onCreate(savedInstanceState); setContentView(R.layout.activity_main); Button button1 = (Button) findViewById(R.id.button); button1.setOnClickListener(this ); } @Override public void onClick (View v) { switch (v.getId()){ case R.id.button: break ; default : break ; } } }
上面代码在IDE中提示由于switch
语句标签太少,应该用if
替换,而且在Android Gradle8.0版本中,资源ID默认非final,所有应该避免在switch case
使用。修改后的
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 public class MainActivity extends AppCompatActivity implements View.OnClickListener{ @Override protected void onCreate(@Nullable Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); Button button1 = (Button) findViewById(R.id.button); button1.setOnClickListener(this); } @Override public void onClick(View v) { if (v.getId()==R.id.button){ // 添加逻辑 }else{ // 添加逻辑 } } }
两种方式都可以实现对按钮点击事件的监听
EditText EditText是程序用于和用户进行交互的另一个重要控件,它允许用户在控件里输入和编辑内容,并可以在程序中对这些内容进行处理。 修改activity_main.xml中的代码
1 2 3 4 <EditText android:id="@+id/edit_text" android:layout_width="match_parent" android:layout_height="wrap_content"/
这就是使用XML来编写界面,完全不用借助可视化工具来实现。运行程序,即可看到EditText的显示,我们可以在里面输入内容。 输入框提示文字,修改activity_main.xml:
1 2 3 4 5 <EditText android:id="@+id/edit_text" android:layout_width="match_parent" android:layout_height="wrap_content" android:hint="Type something here"/>
这里android:hint属性指定了一段提示性文本,当我们输入任何内容时,这段文本就会自动消失。 随内容不断增多,EditText会不断拉长,由于EditText的高度指定的是wrap_content,所以它总能包含住里面的内容,但是当输入的内容过多时,界面就会变的难看,我们可以使用android:maxLines属性来解决这个问题,修改activity_main.xml:
1 2 3 4 5 6 <EditText android:id="@+id/edit_text" android:layout_width="match_parent" android:layout_height="wrap_content" android:hint="Type something here" android:maxLines="2"/>
这里指定了ExitText的最大行数为两行,当输入的内容超过两行时,文本就会向上滚动,而EditText不会继续拉伸。 还可以结合使用EditText与Button实现一些功能,如通过点击按钮来获取EditText中输入的内容。修改MainActivity中的代码
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 public class MainActivity extends AppCompatActivity implements View.OnClickListener{ private EditText editText; @Override protected void onCreate(@Nullable Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); Button button1 = (Button) findViewById(R.id.button); button1.setOnClickListener(this); editText = (EditText) findViewById(R.id.edit_text); } @Override public void onClick(View v) { if (v.getId()==R.id.button){ String inputText = editText.getText().toString(); Toast.makeText(MainActivity.this,inputText,Toast.LENGTH_SHORT).show(); }else{ // 添加逻辑 } } }
通过findViewById()方法得到EditText的实例,然后在按钮的点击事件里调用EditText的getText()方法获取到输入的内容,再调用toString()方法转为字符串,最后用Toast将输入的内容显示出来。
ImageView ImageView是用于在界面上展示图片的一个控件,它可以让程序更加丰富多彩。我们需要提前准备一些图片,图片通常放在以”drawable”开头的目录下。我们项目中已经有一个drawable目录了,但是还没有指定具体的分辨率,所以一般不使用它来放置图片。我们在res目录下新建一个drawable-xhdpi目录,放置两张图片img_1.png和img_2.png。修改activity_xml:
1 2 3 4 5 6 7 8 <ImageView android:id="@+id/image_view" android:layout_width="wrap_content" android:layout_height="wrap_content" android:src="@drawable/img_1"/> ```   这里使用android:src属性给ImageView指定了一张图片。由于图片的宽和高都是未知,所以将ImageView的宽和高都设定为wrap_content。   我们还可以在程序中通过代码动态地更改ImageView中的图片,然后修改MainActivity的代码:
public class MainActivity extends AppCompatActivity implements View.OnClickListener{
private EditText editText;
private ImageView imageView;
@Override
protected void onCreate(@Nullable Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
Button button1 = (Button) findViewById(R.id.button);
button1.setOnClickListener(this);
editText = (EditText) findViewById(R.id.edit_text);
imageView = (ImageView) findViewById(R.id.image_view);
}
@Override
public void onClick(View v) {
if (v.getId()==R.id.button){
imageView.setImageResource(R.drawable.img_2);
}else{
// 添加逻辑
}
}
}
1 2 3   在按钮点击事件里,通过调用ImageView的setImageResource()方法将显示的图片改成了img_2,运行程序,点击按钮就可以看到ImageView中显示的图片改变了。 ## ProgressBar   ProgressBar用于在界面上显示一个进度条,表示我们的程序正在加载一些数据。用法很简单:
1 2   重新运行程序,屏幕中会有一个圆形进度条在旋转。为了能让进度条在数据加载完成后消失,我们要用到一个属性:Android控件的可见属性。所有的Android控件都具有这个属性,可以通过android:visibility进行指定,可选值有3种:visible、invisible和gone。visible表示控件是可见的,这个值是默认值,不指定android:visibility时,控件都是可见的。invisible表示控件不可见,但它还占据着原来位置的大小,可以理解为是控件变成透明状态了。gone表示控件不仅不可见,而且不再占据任何屏幕空间。我们可以通过代码来设置控件的可见性,使用的是setVisibility()方法,可以传入View.VISIBLE、View.INVISIBLE和View.GONE这三种值。   我们通过点击按钮让进度条消失:
public class MainActivity extends AppCompatActivity implements View.OnClickListener{
private EditText editText;
private ImageView imageView;
private ProgressBar progressBar;
@Override
protected void onCreate(@Nullable Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
Button button1 = (Button) findViewById(R.id.button);
button1.setOnClickListener(this);
editText = (EditText) findViewById(R.id.edit_text);
imageView = (ImageView) findViewById(R.id.image_view);
progressBar = (ProgressBar) findViewById(R.id.progress_bar);
}
@Override
public void onClick(View v) {
if (v.getId()==R.id.button){
if (progressBar.getVisibility()==View.GONE){
progressBar.setVisibility(View.VISIBLE);
}else {
progressBar.setVisibility(View.GONE);
}
}else{
// 添加逻辑
}
}
}
1 2   在按钮点击事件中,我们通过getVisibility()方法来判断ProgressBar是否可见,如果可见就隐藏掉,如果不可见就将ProgressBar显示出来。重新运行程序,不断点击按钮,就会看到进度条会在显示和隐藏之间来回切换。   还可以给ProgressBar指定不同的样式,刚刚看到的是圆形进度条,通过style属性可以将它设置成水平进度条,修改activity_main.xml中的代码:
1 2 3 4 5 6 7 8 9 10 11 12   指定为水平进度条之后,通过android:max属性给进度条设置一个最大值,然后在代码中动态地更改进度条的进度。修改MainActivity中的代码: ```java @Override public void onClick(View v) { if (v.getId()==R.id.button){ int progress=progressBar.getProgress(); progress=progress+10; progressBar.setProgress(progress); }else{ // 添加逻辑 } }
每点击一次按钮,我们就获取当前进度,然后在现有的进度上加10作为更新后的进度。
AlertDialog AlertDialog可以在当前界面弹出一个对话框,这个对话框是置顶于所有界面元素之上的,能够屏蔽掉其他控件的交互功能,因此,AlertDialog一般用于提示一些非常重要的内容或者警告信息。 比如为例防止用户删除重要内容,在删除前弹出一个确认对话框。修改MainActivity中的代码:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 @Override public void onClick (View v) { if (v.getId()==R.id.button){ AlertDialog.Builder dialog = new AlertDialog .Builder(MainActivity.this ); dialog.setTitle("This is Dialog" ); dialog.setMessage("Something important" ); dialog.setCancelable(false ); dialog.setPositiveButton("OK" , new DialogInterface .OnClickListener() { @Override public void onClick (DialogInterface dialog, int which) { } }); dialog.setNegativeButton("Cancel" , new DialogInterface .OnClickListener() { @Override public void onClick (DialogInterface dialog, int which) { } }); dialog.show(); }else { } }
首先通过AlertDialog.Builder创建一个AlertDialog的实例,然后可以为这个对话框设置标题、内容、可否取消等特性,接下来调用setPositiveButton()方法为对话框设置确定按钮的点击事件,调用setNegativeButton()方法设置取消按钮的点击事件,最后调用show()方法将对话框显示出来。
ProgressDialog ProgressDialog和AlertDialog有点类似,都可以在界面上弹出一个对话框,都能够屏蔽掉其 他控件的交互能力。不同的是,ProgressDialog会在对话框中显示一个进度条,一般用于表示当前操作比较耗时,让用户耐心等待。用法也和AlertDialog相似,修改MainActivity代码:
1 2 3 4 5 6 7 8 9 10 11 12 @Override public void onClick(View v) { if (v.getId()==R.id.button){ ProgressDialog progressDialog = new ProgressDialog(MainActivity.this); progressDialog.setTitle("This is ProgressDialog"); progressDialog.setMessage("Loading..."); progressDialog.setCancelable(true); progressDialog.show(); }else{ // 添加逻辑 } }
这里也是先构建ProgressDialog对象,然后设置标题,内容,可否取消等属性,最后通过show()方法将ProgressDialog显示出来。 注意,如果在setCancelable()中传入了false,表示ProgressDialog是不能通过Back键取消掉的,这时就一定要在代码中做好控制,当数据加载完成后必须调用ProgressDialog的dismiss()方法来关闭对话框,否则ProgressDialog将会一直存在。
四种布局 新建一个UILayoutTest项目,让Android Studio自动帮我们创建活动,活动名和布局名都是用默认值。
线性布局 LinearLayout又称为线性布局,这个布局会将它所包含的控件在线性方向上依次排列。线性排列有两种方向,垂直方向排列和水平方向排列。而上面的案例中都是通过android:orientation属性指定了排列方向是vertical,如果指定的是horizontal,控件就会在水平方向上排列了。修改activity_main.xml中代码:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 <LinearLayout xmlns:android ="http://schemas.android.com/apk/res/android" android:orientation ="vertical" android:layout_width ="match_parent" android:layout_height ="match_parent" > <Button android:id ="@+id/button1" android:layout_width ="wrap_content" android:layout_height ="wrap_content" android:text ="Button1" /> <Button android:id ="@+id/button2" android:layout_width ="wrap_content" android:layout_height ="wrap_content" android:text ="Button2" /> <Button android:id ="@+id/button3" android:layout_width ="wrap_content" android:layout_height ="wrap_content" android:text ="Button3" /> </LinearLayout >
然后我们修改LinearLayout的排列方向:
1 2 3 4 5 <LinearLayout xmlns:android ="http://schemas.android.com/apk/res/android" android:orientation ="horizontal" //排列方向 android:layout_width ="match_parent" android:layout_height ="match_parent" > ...
如果我们不指定android:orientation属性的值,默认排列方向就是horizontal。 注意:如果LinearLayout的排列方向是horizontal,内部的控件就绝不能将宽度指定为match_parent,因为这样的话,单独一个控件就会将整个水平方向占满,其他控件就没有可放置的位置了。同样,如果LinearLayout的排列方向是vetical,内部控件就不能将高度指定为match_parent。 首先看android:layout_gravity属性,它和android:gravity属性相似,android:gravity用于指定文字在控件中的对齐方式,而android:layout_gravity用于指定控件在布局中的对齐方式。需要注意的是,当LinearLayout的排列方式是horizontal时,只有垂直方向的对齐方式才会生效,因为水平方向的长度是不固定的,每添加一个控件,水平方向上的长度都会改变,因而无法指定该方向上的对齐方式。同样,当LinearLayout的排列方向是vertical时,只有水平方向的对齐方式才会生效。修改activity_main.xml中的代码:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" android:orientation="horizontal" android:layout_width="match_parent" android:layout_height="match_parent"> <Button android:id="@+id/button1" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_gravity="top" android:text="Button1" /> <Button android:id="@+id/button2" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_gravity="center_vertical" android:text="Button2" /> <Button android:id="@+id/button3" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_gravity="bottom" android:text="Button3" /> </LinearLayout>
由于目前LinearLayout的排列方向是horizontal,因此我们只能指定垂直方向上的排列方向。 LineatLayout另一个重要属性——android:layout_weight这个属性允许我们使用比例的方式来指定控件的大小,它在手机屏幕的适配性方面可以起到非常重要的作用。比如我们编写一个消息发送界面,需要文本编辑框和一个发送按钮,修改activity_main.xml中的代码:
1 2 3 4 5 6 7 8 9 10 11 12 13 <EditText android:id="@+id/input_message" android:layout_width="0dp" android:layout_height="wrap_content" tools:ignore="Suspicious0dp" android:layout_weight="3" android:hint="Type somethind"/> <Button android:id="@+id/send" android:layout_width="0dp" android:layout_height="wrap_content" android:layout_weight="2" android:text="Send"/>
这里将EditText和Button的宽度都指定成了0dp,但是我们使用了android:layout_weight属性,此时控件的宽度就不由android:layout_width来决定,这里指定为0dp是一种规范写法。dp是Android中指定控件大小、间距等属性的单位。 在EditText和Button里都将android:layout_weight属性的值都指定为1,表示EditText和Button将在水平方向平分宽度。其原理就是系统会先把LinearLayout下所有的控件指定的Layout_weight值相加,得到一个总值,然后每个控件所占大小的比例就是用该控件的layout_weight值除以刚才算出的总值。因此如果想让EditText占据屏幕宽度的3/5,Button占据屏幕宽度的2/5,只需将EditText的layout_weight改成3,Button的layout_weight改成2就可以了。 还可以通过指定部分控件的layout_weight值来实现更好的效果。修改activity_main.xml代码:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:tools="http://schemas.android.com/tools" android:orientation="horizontal" android:layout_width="match_parent" android:layout_height="match_parent"> <EditText android:id="@+id/input_message" android:layout_width="0dp" android:layout_height="wrap_content" tools:ignore="Suspicious0dp" android:layout_weight="1" android:hint="Type somethind"/> <Button android:id="@+id/send" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="Send"/> </LinearLayout>
这里仅指定了EditText的android:layout_weight属性,并将Button的宽度改回了wrap_content。表示Button的宽度仍然按照wrap_content来计算,而EditText则会沾满屏幕所有剩余空间。这种方式在屏幕适配方面效果比较好。
相对布局 RelativeLayout又称为相对布局,也是一种常用布局。和LinearLayout的排列规则不同,RelativeLayout显得更加随意,它可以通过相对定位的方式让控件出现在布局的任何位置。正是如此,RelativeLayout中的属性很多,但是这些属性都是有规律可循的,修改activity_main.xml中的代码:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 <?xml version="1.0" encoding="utf-8" ?> <RelativeLayout xmlns:android ="http://schemas.android.com/apk/res/android" android:layout_width ="match_parent" android:layout_height ="match_parent" > <Button android:id ="@+id/button1" android:layout_width ="wrap_content" android:layout_height ="wrap_content" android:layout_alignParentLeft ="true" android:layout_alignParentTop ="true" android:text ="Button 1" /> <Button android:id ="@+id/button2" android:layout_width ="wrap_content" android:layout_height ="wrap_content" android:layout_alignParentRight ="true" android:layout_alignParentTop ="true" android:text ="Button 2" /> <Button android:id ="@+id/button3" android:layout_width ="wrap_content" android:layout_height ="wrap_content" android:layout_centerInParent ="true" android:text ="Button 3" /> <Button android:id ="@+id/button4" android:layout_width ="wrap_content" android:layout_height ="wrap_content" android:layout_alignParentBottom ="true" android:layout_alignParentLeft ="true" android:text ="Button 4" /> <Button android:id ="@+id/button5" android:layout_width ="wrap_content" android:layout_height ="wrap_content" android:layout_alignParentBottom ="true" android:layout_alignParentRight ="true" android:text ="Button 5" /> </RelativeLayout >
效果: 上面例子中每个控件都是相对于父布局进行定位的,我们可以让控件相对于控件进行定位,修改activity_main.xml中的代码:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 <?xml version="1.0" encoding="utf-8" ?> <RelativeLayout xmlns:android ="http://schemas.android.com/apk/res/android" android:layout_width ="match_parent" android:layout_height ="match_parent" > <Button android:id ="@+id/button3" android:layout_width ="wrap_content" android:layout_height ="wrap_content" android:layout_centerInParent ="true" android:text ="Button 3" /> <Button android:id ="@+id/button1" android:layout_width ="wrap_content" android:layout_height ="wrap_content" android:layout_above ="@id/button3" android:layout_toLeftOf ="@id/button3" android:text ="Button 1" /> <Button android:id ="@+id/button2" android:layout_width ="wrap_content" android:layout_height ="wrap_content" android:layout_above ="@+id/button3" android:layout_toRightOf ="@id/button3" android:text ="Button 2" /> <Button android:id ="@+id/button4" android:layout_width ="wrap_content" android:layout_height ="wrap_content" android:layout_below ="@id/button3" android:layout_toLeftOf ="@id/button3" android:text ="Button 4" /> <Button android:id ="@+id/button5" android:layout_width ="wrap_content" android:layout_height ="wrap_content" android:layout_below ="@id/button3" android:layout_toRightOf ="@id/button3" android:text ="Button 5" /> </RelativeLayout >
要注意的是,当一个控件去引用另一个控件的id时,该控件一定要定义在引用控件的后面,不然会出现找不到id的情况。 RelativeLayout中还有另外一组相对于控件进行定位的属性,android:layout_alignLeft表示让一个控件的左边缘和另一个控件的左边缘对齐,android:layout_alignRight表示让一个控件的右边缘和另一个控件的右边缘对齐。还有android:layout_alignTop和android:layout_alignBotton道理相同。
帧布局 FrameLayout又称作帧布局,它相比前两种布局简单很多,应用场景也少很多。这种布局没有方便的定位方式,所有的控件都会默认在布局左上角。修改activity_main.xml中的代码:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 <?xml version="1.0" encoding="utf-8" ?> <FrameLayout xmlns:android ="http://schemas.android.com/apk/res/android" android:layout_width ="match_parent" android:layout_height ="match_parent" > <TextView android:id ="@+id/text_view" android:layout_width ="wrap_content" android:layout_height ="wrap_content" android:text ="This is TextView" /> <ImageView android:id ="@+id/image_view" android:layout_width ="wrap_content" android:layout_height ="wrap_content" android:src ="@mipmap/ic_launcher" /> </FrameLayout >
FrameLayout中只是放置了一个TextView和一个ImageView,这里我们直接使用了@mipmap来访问ic_launcher这张图。 可以看到文字和图片都是位于布局左上角。由于ImageView是在TextView之后添加的,因此图片压在了文字的上面。 除了这种默认效果,我们还可以使用layout_gravity属性来指定控件在布局中的对齐方式,这和LinearLayout中的用法是相似的。修改activity_main.xml中的代码:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 <?xml version="1.0" encoding="utf-8" ?> <FrameLayout xmlns:android ="http://schemas.android.com/apk/res/android" android:layout_width ="match_parent" android:layout_height ="match_parent" > <TextView android:id ="@+id/text_view" android:layout_width ="wrap_content" android:layout_height ="wrap_content" android:layout_gravity ="left" android:text ="This is TextView" /> <ImageView android:id ="@+id/image_view" android:layout_width ="wrap_content" android:layout_height ="wrap_content" android:layout_gravity ="right" android:src ="@mipmap/ic_launcher" /> </FrameLayout >
指定TextView居左对齐,ImageView居右对齐。 由于FrameLayout的定位方式欠缺,它的应用场景也比较少。
百分比布局 前面的布局中只有LinearLayout支持使用layout_weight属性来实现按比例指定大小的功能,其他两种都不支持。如果想要用RelativeLayout来实现让两个按钮评分布局宽度的效果,则比较困难。 为此,Android引入了新的布局方式来解决这个问题——百分比布局。这种布局中,不再使用wrap_content、match_parent等方式指定控件的大小了,而是允许直接直指定控件所占的百分比,这样可以轻松实现平分布局甚至任意比例分割布局的效果。 百分比布局提供了PercentFrameLayout和PercentRelativeLayout这两个全新的布局。Android将百分比布局定义在了suport库中,我们只需要在项目的build.gradle中添加百分比布局库的依赖,就能保证百分比布局在Android所有系统版本上的兼容性了。 打开app/build.gradle文件,在dependencies闭包中添加如下内容:
1 2 3 dependencies { implementation ("androidx.percentlayout:percentlayout:1.0.0") }
每当修改了任何gradle文件时,Android Studio都会弹出提示,需要我们再次同步才能使项目正常工作,点击Sync Now
即可。修改activity_main.xml中的代码:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 <?xml version="1.0" encoding="utf-8"?> <androidx.constraintlayout.widget.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" xmlns:app="http://schemas.android.com/apk/res-auto"> <!-- Button 1 --> <Button android:id="@+id/button1" android:text="Button1" android:layout_width="0dp" android:layout_height="0dp" app:layout_constraintTop_toTopOf="parent" app:layout_constraintStart_toStartOf="parent" app:layout_constraintEnd_toStartOf="@id/button2" app:layout_constraintBottom_toTopOf="@id/button3" /> <!-- Button 2 --> <Button android:id="@+id/button2" android:text="Button2" android:layout_width="0dp" android:layout_height="0dp" app:layout_constraintTop_toTopOf="parent" app:layout_constraintEnd_toEndOf="parent" app:layout_constraintStart_toEndOf="@id/button1" app:layout_constraintBottom_toTopOf="@id/button4" /> <!-- Button 3 --> <Button android:id="@+id/button3" android:text="Button3" android:layout_width="0dp" android:layout_height="0dp" app:layout_constraintStart_toStartOf="parent" app:layout_constraintBottom_toBottomOf="parent" app:layout_constraintEnd_toStartOf="@id/button4" app:layout_constraintTop_toBottomOf="@id/button1" /> <!-- Button 4 --> <Button android:id="@+id/button4" android:text="Button4" android:layout_width="0dp" android:layout_height="0dp" app:layout_constraintEnd_toEndOf="parent" app:layout_constraintBottom_toBottomOf="parent" app:layout_constraintStart_toEndOf="@id/button3" app:layout_constraintTop_toBottomOf="@id/button2" /> </androidx.constraintlayout.widget.ConstraintLayout> ``` 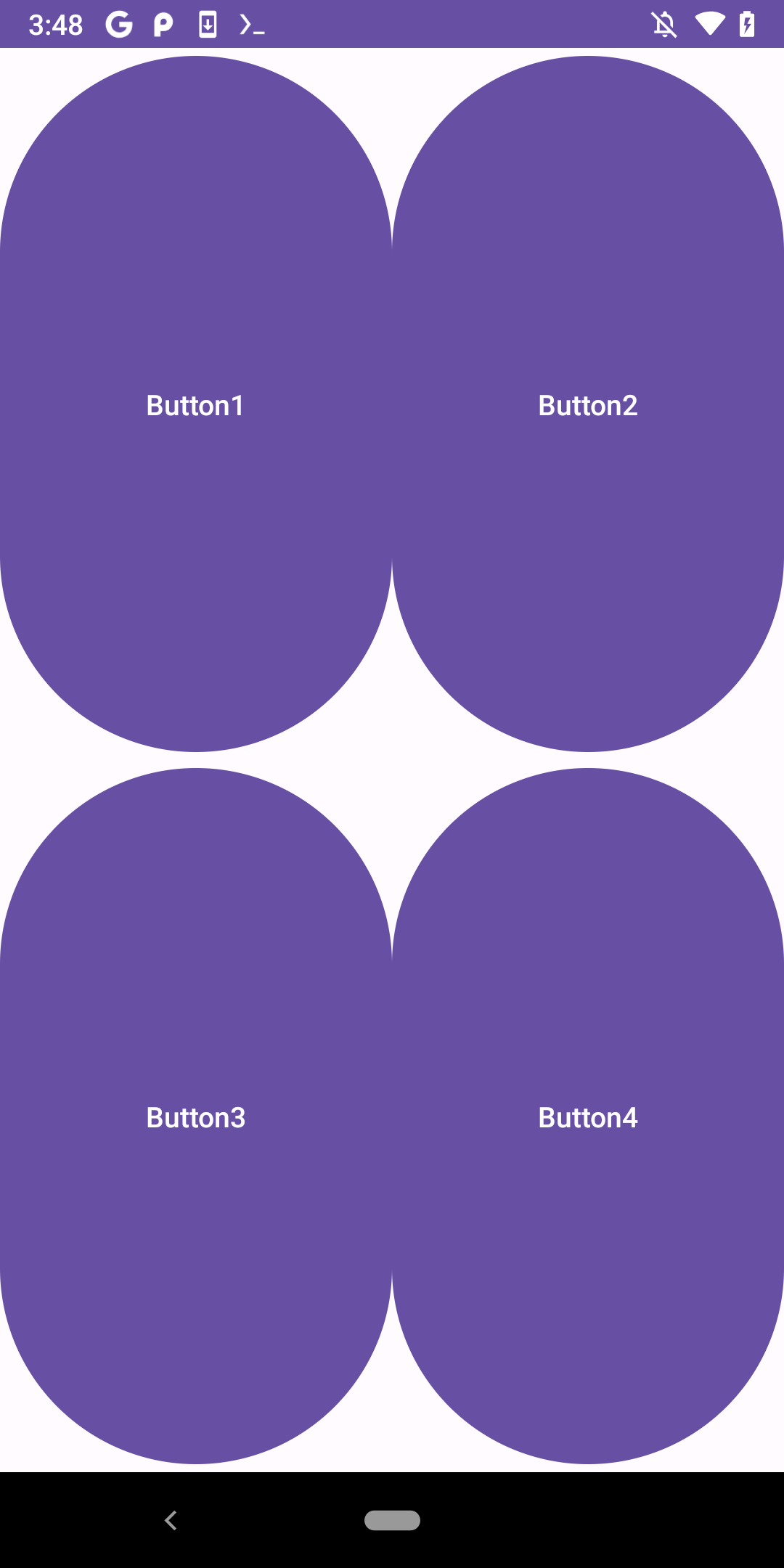 # 创建自定义控件 控件和布局的继承结构 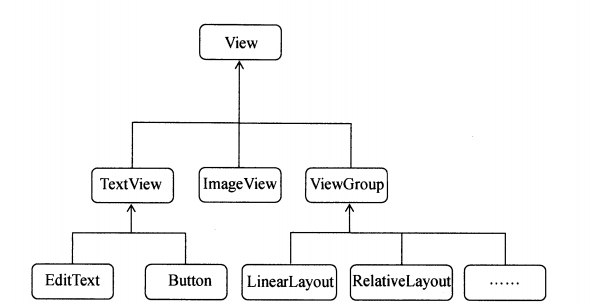   所用的控件都是直接或间接继承自View的,所用的布局都是直接或间接继承自ViewGroup的。View是Android中最基本的一种UI组件,它可以在屏幕上绘制一块矩形区域,并能影响这块区域的各种事件,我们使用的各种控件其实就是在View的基础之上添加了各自特有的功能。而ViewGroup则是一种特殊的View,它包含了很多子View和子ViewGroup,是一个放置控件和布局的容器。   创建一个UICustomViews项目。 ## 引入布局   在iPhone中,几乎每个应用的界面顶部都会有一个标题栏,标题栏上会有一到两个按钮可用于返回或其他操作。这里我们创建自定义标题栏。   只需要加入两个Button和一个TextView,然后在布局中摆放好就可以了。 为了减少代码重复,可以使用引入布局的方式来解决这个问题,新建一个布局title.xml:
<Button
android:id="@+id/title_back"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_gravity="top"
android:layout_margin="5dp"
android:text="Back"
android:textColor="#fff" />
<TextView
android:id="@+id/title_text"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_gravity="top"
android:layout_weight="1"
android:gravity="center"
android:text="Title Text"
android:textColor="#000"
android:textSize="24sp"/>
<Button
android:id="@+id/title_edit"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_gravity="top"
android:layout_margin="5dp"
android:text="Edit"
android:textColor="#fff"
/>
1 2 3 4 5 6 7 8 9   在这里使用了android:layout_margin属性,它可以指定控件在上下左右方向上偏移的距离,也可以用android:layout_marginLeft或android:layout_marginTop等属性单独指定控件在某个方向上偏移的距离。   标题栏布局编写完成,剩下就是在程序中使用标题栏,修改activity_main.xml中代码: ```xml <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" android:layout_width="match_parent" android:layout_height="match_parent"> <include layout="@layout/title"/> </LinearLayout>
隐藏系统自带标题
1 2 3 4 5 6 7 8 9 @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); ActionBar actionbar= getSupportActionBar(); if (actionbar!= null){ actionbar.hide(); } }
通过调用getSupportActionBar()方法来获得ActionBar的实例,然后调用ActionBar的hide()方法将标题隐藏起来。
## 创建自定义控件
新建TitleLayout继承自LinearLayout,让它成为我们自定义的标题栏控件:
1 2 3 4 5 6 public class TitleLayout extends LinearLayout { public TitleLayout(Context context, @Nullable AttributeSet attrs) { super(context, attrs); LayoutInflater.from(context).inflate(R.layout.title,this); } }
我们重写了LinearLayout中带有两个参数的构造函数,在布局中引入TitleLayout控件就会调用这个构造函数。然后在构造函数中需要对标题栏布局进行动态加载,借助LayoutInflater来实现。通过LayoutInflater的from()方法可以构建出一个LayoutInflater对象,然后调用inflate()方法动态加载一个布局文件,inflate()方法接收两个参数,第一个参数是要加载布局文件的id这里我们传入R.id.title,第二个参数给加载好的布局再添加一个父布局,这里我们想要指定为TitleLayout,传入this。
在布局文件中添加这个自定义控件,修改activity_main.xml中的代码:
1 2 3 4 5 6 7 8 9 <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent"> <com.example.uicustomviews.TitleLayout tools:ignore="MissingClass" android:layout_height="match_parent" android:layout_width="wrap_content"/> </LinearLayout>
添加自定义控件和添加普通控件的方式基本一样,只不过在添加自定义控件时,我们要指明控件的完整类名。
为标题栏的按钮注册点击事件,修改TitleLayout中的代码:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 public class TitleLayout extends LinearLayout { public TitleLayout (Context context, @Nullable AttributeSet attrs) { super (context, attrs); LayoutInflater.from(context).inflate(R.layout.title,this ); Button titleedit = (Button) findViewById(R.id.title_edit); Button titleback = (Button) findViewById(R.id.title_back); titleback.setOnClickListener(new OnClickListener () { @Override public void onClick (View v) { ((Activity)getContext()).finish(); } }); titleedit.setOnClickListener(new OnClickListener () { @Override public void onClick (View v) { Toast.makeText(getContext(),"You cliclk Edit button" ,Toast.LENGTH_SHORT).show(); } }); } }
先通过findViewById()方法得到按钮实例然后调用setOnClickListener()方法给两个按钮注册点击事件,当点击返回按钮时,销毁当前的活动,当点击编辑按钮时弹出一段文本。
这样每当在一个布局中引入TitleLayout时,返回按钮和编辑按钮的点击事件就已经自动实现好了。
# ListView
由于手机屏幕空间都比较有限,能够一次性在屏幕上显示的内容不多,当我们的程序中有大量的数据需要展示时,就可以借助ListView来实现。ListView允许用户通过手指上下滑动的方式将屏幕外的数据滚动到屏幕内,同时屏幕上原有的数据则会滚动出屏幕。
## ListView的简单用法
先新建一个ListViewTest项目,让Android Studio自动帮我们创建好活动。修改activity_main.xml中的代码:
1 2 3 4 5 6 7 8 9 10 11 12 13 <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:app="http://schemas.android.com/apk/res-auto" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" tools:context=".MainActivity"> <ListView android:id="@+id/list_view" android:layout_width="match_parent" android:layout_height="match_parent" /> </LinearLayout>
修改MainActivity中的代码:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 public class MainActivity extends AppCompatActivity { private String[] data = {"Apple","Banana","Orange","Watermelon", "Pear","Grape","Pineapple","Strawberry","Cherry","Mango", "Apple","Banana","Orange","Watermelon", "Pear","Grape","Pineapple","Strawberry","Cherry","Mango"}; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); ArrayAdapter<String> adapter = new ArrayAdapter<String>( MainActivity.this, android.R.layout.simple_list_item_1,data ); ListView listView= (ListView) findViewById(R.id.list_view); listView.setAdapter(adapter); } }
我们用了data数组来测试,里面包含了很多水果的名称。不过数组中的数据是无法直接传递给ListView的,我们还需要借助适配器来完成。Android中提供了很多适配器的实现类,其中ArrayAdapter较好用。它可以通过泛型来指定要适配的数据类型,然后在构造函数中把要适配的数据传入。ArrayAdapter有多个构造函数的重载,根据实际情况选择最合适的一种。ArrayAdapter的构造函数中依次传入当前上下文、ListView子项布局的id,以及要适配的数据。我们使用了android.R.layout.simple_list_item_1作为ListView子项布局的id,这是一个Android内置的布局文件,里面只有一个TextView,用于简单地显示一段文本。
最后调用ListView的setAdapter()方法,将构建好的适配器对象传递进去,ListView和数据之间的关联就建立完成了。
## 定制ListView的界面
首先准备一组图片,分别对应上面提供的每一种水果,让这些水果名称的旁边都有一个图样。(资源文件名只能包含小写字母,不能包含大写字母)。
定义实体类,作为ListView适配器的适配类型。新建类Fruit:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 public class Fruit { private String name; private int imageId; public Fruit(String name,int imageId){ this.name=name; this.imageId=imageId; } public String getName(){ return name; } public int getImageId(){ return imageId; } }
Fruit类中只有两个字段,name表示水果的名字,imageId表示水果对应图片的资源id。
为ListView的子项指定我们自定义的布局,在layout目录下新建fruit_item.xml:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" android:layout_width="match_parent" android:layout_height="match_parent"> <ListView android:id="@+id/fruit_image" android:layout_width="wrap_content" android:layout_height="wrap_content"/> <TextView android:id="@+id/fruit_name" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_gravity="center" android:layout_marginLeft="10dp"/> </LinearLayout>
这个布局里,定义了一个ImageView用于显示水果的图片,又定义了一个TextView用于显示水果名称,让TextView在垂直方向上居中显示。
然后创建自定义适配器,这个适配器继承自ArrayAdapter,并将泛型指定为Fruit类。新建类FruitAdapter:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 public class FruitAdapter extends ArrayAdapter<Fruit> { private int resourceId; public FruitAdapter(@NonNull Context context, int textViewResourceId, @NonNull List<Fruit> objects) { super(context,textViewResourceId, objects); resourceId=textViewResourceId; } @NonNull @Override public View getView(int position, @Nullable View convertView, @NonNull ViewGroup parent) { Fruit fruit = getItem(position); //获取当前项的Fruit实例 View view = LayoutInflater.from(getContext()).inflate(resourceId,parent,false); ImageView fruitImage = (ImageView) view.findViewById(R.id.fruit_image); TextView fruitName = (TextView) view.findViewById(R.id.fruit_name); fruitImage.setImageResource(fruit.getImageId()); fruitName.setText(fruit.getName()); return view; } }
FruitAdapter重写了父类的一组构造函数,用于将上下文、ListView子项布局的id和数据都传递过来。另外又重写了getView()方法,这个方法在每个子项被滚动到屏幕内的时候会被调用。在getView()方法中,首先通过getItem()方法得到当前项的Fruit实例,然后使用LayoutInflater来为这个子项加载我们传入的布局。
这里LayoutInflater的inflate()方法,接收三个参数,前两个参数已经知道了,第三个参数指定成了false,表示只让我们在父布局中声明的layout属性生效,但不为这个View添加父布局,因为一旦View有了父布局之后,它就不能再添加到ListView中了。
往下,接下来调用View的findVIewById()方法分别获取到ImageView和TextView的实例,并分别调用他们的setImageResource()和setText()方法来设置显示的图片和文字,最后将布局返回,这样自定义的适配器就完成了。修改MainActivity中的代码:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 public class MainActivity extends AppCompatActivity { private List<Fruit> fruitList = new ArrayList <>(); @Override protected void onCreate (Bundle savedInstanceState) { super .onCreate(savedInstanceState); setContentView(R.layout.activity_main); initFruits(); FruitAdapter adapter = new FruitAdapter (MainActivity.this ,R.layout.fruit_item,fruitList); ListView listView = (ListView) findViewById(R.id.list_view); listView.setAdapter(adapter); } private void initFruits () { for ( int i = 0 ;i < 2 ;i++){ Fruit apple = new Fruit ("Apple" , R.drawable.Apple); fruitList.add(apple); Fruit banana = new Fruit ("banana" ,R.drawable.Banana); fruitList.add(banana); Fruit orange = new Fruit ("orange" ,R.drawable.Orange); fruitList.add(orange); Fruit cherry = new Fruit ("cherry" ,R.drawable.Cherry); fruitList.add(cherry); Fruit grape = new Fruit ("grape" ,R.drawable.Grape); fruitList.add(grape); Fruit mongo = new Fruit ("mongo" ,R.drawable.Mango); fruitList.add(mongo); Fruit peach = new Fruit ("peach" ,R.drawable.Peach); fruitList.add(peach); Fruit pear = new Fruit ("pear" ,R.drawable.Pear); fruitList.add(pear); Fruit strawberry = new Fruit ("strawberry" ,R.drawable.Strawberry); fruitList.add(strawberry); Fruit watermelon= new Fruit ("watermelon" ,R.drawable.Watermelon); fruitList.add(watermelon); } } }
这里添加了一个initFruits()方法,用于初始化所有的水果数据。在Fruit类的构造函数中将水果的名字和对应的id注入,然后把创建好的对象添加到水果列表中。然后用for循环将所有的水果数据添加了两遍,如果只添加一遍,数据还不足以充满整个屏幕。接着在onCreat()方法创建了FruitAdapter对象,将FruitAdapter作为适配器传递给ListView,定制ListView界面完成。
## ListView的点击事件
修改MainActivity的点击事件:
1 2 3 4 5 6 7 8 listView.setOnItemClickListener(new AdapterView.OnItemClickListener() { @Override public void onItemClick(AdapterView<?> parent, View view, int position, long id) { Fruit fruit = fruitList.get(position); Toast.makeText(MainActivity.this,fruit.getName(),Toast.LENGTH_SHORT).show(); } }); }
使用setOnItemClickListener()方法为ListView注册了一个监听器,当用户点击了ListView中任何一个子项时,就会回调onItemClick()方法。在这个方法中通过position参数来判断用户点击的是哪一个子项,然后获取到相应的水果,并通过Toast将水果名字显示出来。
# 滚动控件RecyclerView
可以说是一个增强版的ListView,不仅可以轻松实现和ListView同样的效果,还优化了ListView中存在的各种不足之处。我们先新建一个RecycleView项目。
## RecycleView基本用法
1 2 3 4 5 6 7 8 9 10 11 12 13 <androidx.constraintlayout.widget.ConstraintLayout xmlns:android ="http://schemas.android.com/apk/res/android" xmlns:app ="http://schemas.android.com/apk/res-auto" xmlns:tools ="http://schemas.android.com/tools" android:layout_width ="match_parent" android:layout_height ="match_parent" tools:context =".MainActivity" > <androidx.recyclerview.widget.RecyclerView android:id ="@+id/recycle_view" android:layout_width ="match_parent" android:layout_height ="match_parent" /> </androidx.constraintlayout.widget.ConstraintLayout >
在布局中加入RecycleView也很简单,先为RecycleView指定id,然后将宽度和高度都设置为match_parent,这样Recycle就占满了整个布局的空间。需注意,RecycleView并不是内置在系统SDK中的,所以需要把完整的包路径写出来。
接下来想要使用RecycleView来实现和ListView相同的效果,就要准备一份同样的水果图片。我们直接把从ListViewTest项目中的图片复制过来就可以了,另外也把Fruit类和fruit_item.xml也复制过来。
接下来为RecycleView准备一个适配器,新建FruitAdapter类,让这个适配器继承自RecycleView.Adapter,并将泛型指定为FruitAdapter.ViewHolder。ViewHolder是我们在FruitAdapter中定义的一个内部类:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 public class FruitAdapter extends RecyclerView.Adapter<FruitAdapter.ViewHolder> { private List<Fruit> mFruitList; static class ViewHolder extends RecyclerView.ViewHolder{ ImageView fruitImage; TextView fruitName; public ViewHolder(View view){ super(view); fruitImage=(ImageView) view.findViewById(R.id.fruit_image); fruitName=(TextView) view.findViewById(R.id.fruit_name); } } public FruitAdapter(List<Fruit> fruitList){ mFruitList = fruitList; } @NonNull @Override public FruitAdapter.ViewHolder onCreateViewHolder(@NonNull ViewGroup parent, int viewType) { View view = LayoutInflater.from(parent.getContext()) .inflate(R.layout.fruit_item,parent,false); ViewHolder holder = new ViewHolder(view); return holder; } @Override public void onBindViewHolder(@NonNull FruitAdapter.ViewHolder holder, int position) { Fruit fruit = mFruitList.get(position); holder.fruitImage.setImageResource(fruit.getImageId()); holder.fruitName.setText(fruit.getName()); } @Override public int getItemCount() { return mFruitList.size(); } }
这段代码看上去有点长,但是它要比ListView的适配器要容易理解。我们先定义内部类ViewHolder,ViewHolder要继承自RecycleView.ViewHolder。然后ViewHolder的构造函数中传入一个View参数,这个参数通常就是RecycleView子项的最外层布局,那么我们可以通过findViewById()方法来获取ImageView和TextView的实例。
往下,FruitAdapter中也有一个构造函数,这个方法用于把要展示的数据源传进来,并赋值给一个全局变量mFruitList,后续操作都将在这个数据源的基础上进行。
由于FruitAdapter是继承自RecycleView.Adapter的,就必须重写onCreaterHolder()、onBindViewHolder()和getItemCount()这3个方法。onCreateViewHolder()方法用于创建ViewHolder实例,我们在这个方法中将fruit_item布局加载进来,然后创建一个ViewHolder实例,并把加载出来的布局传入到构造函数中,最后将ViewHolder的实例返回。onBindViewHolder()方法是用于对RecycleView子项的数据进行赋值的,会在每个子项被滚动到屏幕内的时候执行,这里我们通过position参数得到当前项的Fruit实例,然后再将数据设置到ViewHolder的ImageView和TextView当中。getItemCount()就非常简单,它用于告诉RecycleView一共有多少子项,直接返回数据源的长度就可以了。
适配器准备好,开始使用RecycleVIew了,修改MainActivity中的代码。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 public class MainActivity extends AppCompatActivity { private List<Fruit> fruitList = new ArrayList <>(); @Override protected void onCreate (Bundle savedInstanceState) { super .onCreate(savedInstanceState); setContentView(R.layout.activity_main); initFruit(); RecyclerView recyclerView = (RecyclerView) findViewById(R.id.recycle_view); LinearLayoutManager layoutManager = new LinearLayoutManager (this ); recyclerView.setLayoutManager(layoutManager); FruitAdapter adapter = new FruitAdapter (fruitList); recyclerView.setAdapter(adapter); } private void initFruit () { for (int i = 0 ;i<2 ;i++){ Fruit apple = new Fruit ("Apple" , R.drawable.apple); fruitList.add(apple); Fruit banana = new Fruit ("banana" ,R.drawable.banana); fruitList.add(banana); Fruit orange = new Fruit ("orange" ,R.drawable.orange); fruitList.add(orange); Fruit cherry = new Fruit ("cherry" ,R.drawable.cherry); fruitList.add(cherry); Fruit grape = new Fruit ("grape" ,R.drawable.grape); fruitList.add(grape); Fruit mongo = new Fruit ("mongo" ,R.drawable.mango); fruitList.add(mongo); Fruit peach = new Fruit ("peach" ,R.drawable.peach); fruitList.add(peach); Fruit pear = new Fruit ("pear" ,R.drawable.pear); fruitList.add(pear); Fruit watermelon= new Fruit ("watermelon" ,R.drawable.watermelon); fruitList.add(watermelon); } } }